Not a member of Pastebin yet? Sign Up, it unlocks many cool features! Phoenix OS is an Android OS built for the big screen, you can have the best of both worlds from your phone and PC. Notification center Click the icon on the low right to call out the notification center, in which you can manage notifications in bulk. OSG for Android need to use a Level 8 or newer Native ABI and a 2.2 or newer Android version. It's possible to use Android 2.1 devices succesfully but it's not recommended. Older Android versions are not supported. There are specific device models with a bug that need the.
To properly setup an environment for Android development using OpenSceneGraph, we need the following hardware and software:
- Android Tablet – Acer Iconia Tab A510 (Tegra 3 Processor)
- OpenSceneGraph – 3.0.1
- cmake: 2.8+
- g++
- Eclipse Indigo
- Ubuntu 11.04 or Ubuntu 11.10
- Android SDK
- Android NDK
Setup a working Android development environment in Ubuntu:
Open Synaptic Package Manager.
Install package openjdk-6-jdk.
Install the following two packages using either Synaptic or apt-get:
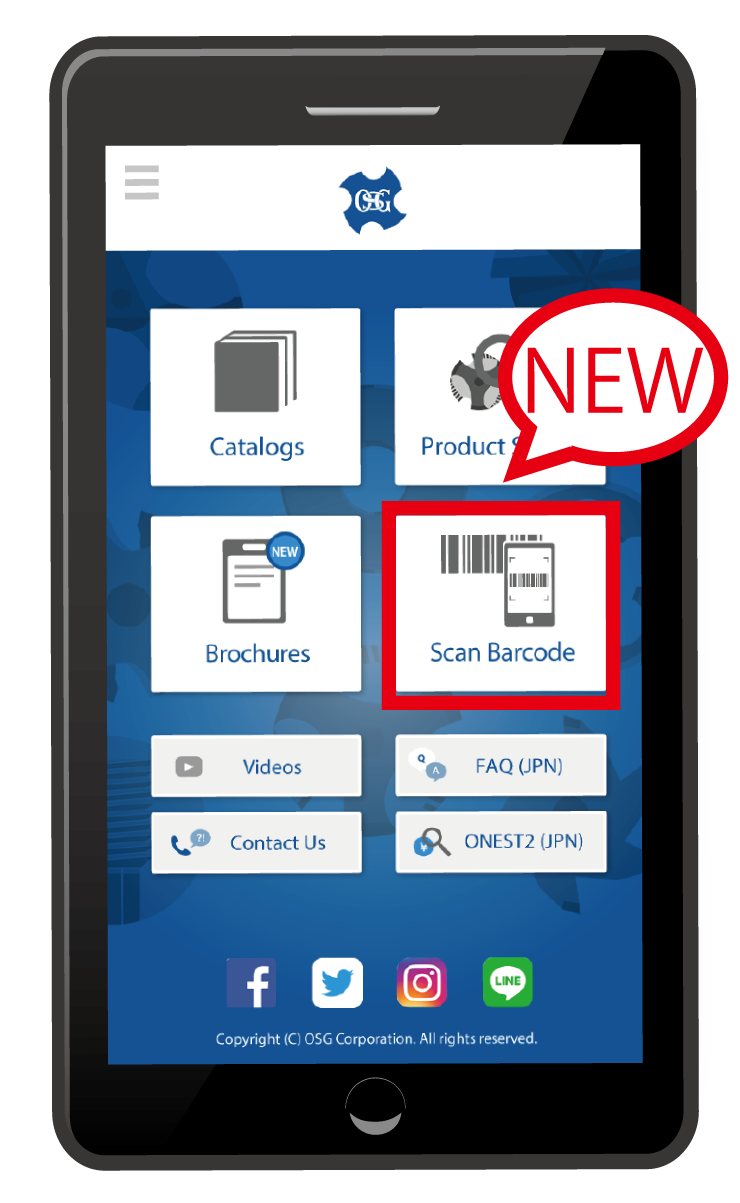
cmake (sudo apt-get install cmake)
g++
Download Eclipse and extract it.
$HOME/Android/eclipse/
Download Android SDK and extract it into
$HOME/Android/android-sdk-linux/
Install ADT-Plugin into Eclipse.
Start Eclipse -> Help -> Install New Software -> in the field ‘Work with:’, fill in “https://dl-ssl.google.com/android/eclipse/”, then press Enter -> check “Developer Tools” -> press Next till everything is installed.
After restarting Eclipse: Window -> Android SDK Manager. Install AT Least Android 2.2 (API 8).(I installed ALL the Android API versions from API 8 to API 15). I installed these APIs to
$HOME/android-sdks/
Extract Android NDK to
$HOME/Android/android-ndk-r8b/
(ndk-r7b and ndk-r7b have been proven to work with OSG)
After everything has been copied to the appropriate directories, we now have a working environment for Android development.
Installing OpenSceneGraph 3.0.1 for Android with OpenGE ES 1.x
Download OpenSceneGraph-3.0.1 (link) and extract it to
$HOME/Android/OpenSceneGraph-3.0.1/
Download the 3rd party dependencies (link) and extract it to
$HOME/Android/OpenSceneGraph-3.0.1/3rdparty/
Add environment variables so that cmake will find the right dependencies, open ~/.bashrc and add the following lines at the end of the file and save it:
export ANDROID_NDK=$HOME/Android/android-ndk-r7b
export ANDROID_SDK=$HOME/android-sdks
Create two folders:
$HOME/Android/OpenSceneGraph-3.0.1/build
$HOME/Android/OpenSceneGraph-3.0.1/osginstall
Open a terminal and change directory to
$HOME/Android/OpenSceneGraph-3.0.1/build
Type in or copy the following command in the terminal and press Enter (the flag -DJ=4 is to specify we have a quad-core processor):
cmake .. -DOSG_BUILD_PLATFORM_ANDROID=ON -DDYNAMIC_OPENTHREADS=OFF -DDYNAMIC_OPENSCENEGRAPH=OFF -DOSG_GL_DISPLAYLISTS_AVAILABLE=OFF -DOSG_GL_MATRICES_AVAILABLE=ON -DOSG_GL_VERTEX_FUNCS_AVAILABLE=ON -DOSG_GL_VERTEX_ARRAY_FUNCS_AVAILABLE=ON -DOSG_GL_FIXED_FUNCTION_AVAILABLE=ON -DOSG_CPP_EXCEPTIONS_AVAILABLE=OFF -DOSG_GL1_AVAILABLE=OFF -DOSG_GL2_AVAILABLE=OFF -DOSG_GL3_AVAILABLE=OFF -DOSG_GLES1_AVAILABLE=ON -DOSG_GLES2_AVAILABLE=OFF -DJ=4 -DCMAKE_INSTALL_PREFIX=$HOME/Android/OpenSceneGraph-3.0.1/osginstall
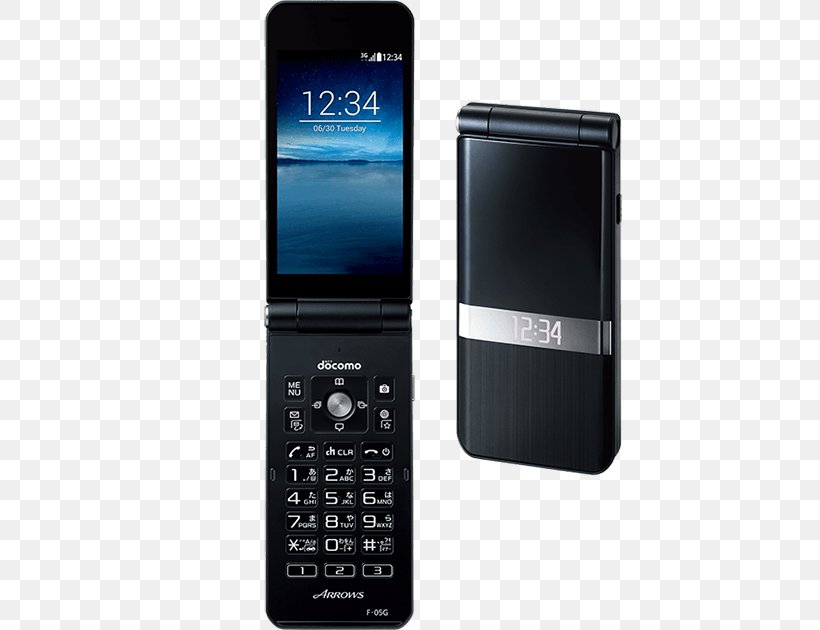
After that, we build it (this step usually takes quite a while):
make
Install libraries and headers into $HOME/Android/OpenSceneGraph-3.0.1/osginstall into the folder obj, lib, and include, by typing:
make install
After this, we have a working environment for developing Android application using OpenSceneGraph.
An Example: creating and build a project
Copy the folder osgAndroidExampleGLES1 from
$HOME/Android/OpenSceneGraph-3.0.1/examples
to your Eclipse workspace
$HOME/workspace/osgAndroidExampleGLES1
Osgearth
Copy the three folders under $HOME/Android/OpenSceneGraph-3.0.1/osginstall, obj, lib, and include to your project folder:
$HOME/workspace/osgAndroidExampleGLES1
Osg Android Download
Start Eclipse -> New -> Project -> Android Project, choose “Create project from existing source” and set the location to $HOME/workspace/osgAndroidExampleGLES1 -> Project Name is “osgAndroidExampleGLES1”, then Next -> build target should be Android 2.2 (API 8) or higher.
In Eclipse project browser, expand the folder jni and open Android.mk in text editor, make the following two changes and save it:
Osg For Android
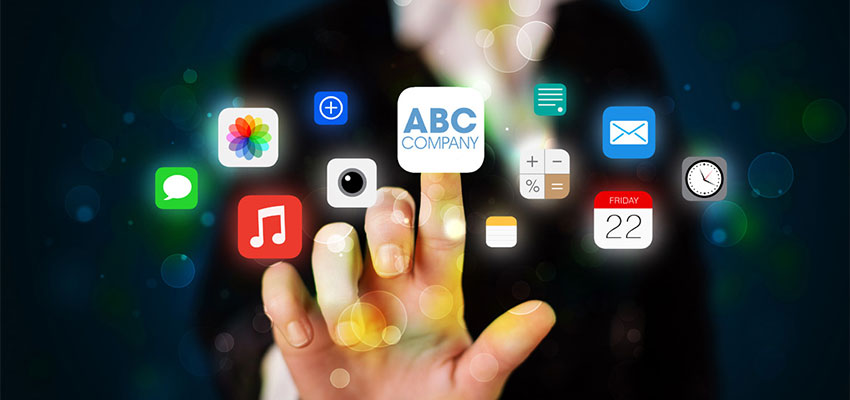
Type
$ANDROID_NDK/ndk-build
Now the native code has been built for the Android OSG Viewer, we can go back to Eclipse and build the project from there.
Openscenegraph Java
A video demonstrating the osgViewer application on the Acer tablet.
* this tutorial was adapted from this link.
I'm trying to run an open scene graph+qt example on my Samsung Galaxy S2 tablet. I'm using Qt 5.8, OpenSceneGraph 3.5.6, Android NDK-r13b and Android 6.0 (API Level 23). FYI - I compiled open scene graph on Android and did not download binaries.
I cannot even get the open scene graph examples to work. Code compiles fine but when I deploy it on my tablet all I see is a blue screen. I have seen this question posted on the forums before but I do not think it has been solved. This is my first post and therefore I cannot post URL links to the previous answers but you can search for the title 'OSG with Qt on Android fails to render anything' on the forums
I have posted my example code below and any help will be appreciated.
Code:
#include 'QTimer'
#include 'QApplication'
#include 'QGridLayout'
#include 'osgViewer/CompositeViewer'
#include 'osgViewer/ViewerEventHandlers'
#include 'osg/PositionAttitudeTransform'
#include 'osgGA/MultiTouchTrackballManipulator'
#include 'osg/ShapeDrawable'
#include 'osgDB/ReadFile'
#include 'osgQt/GraphicsWindowQt'
#include 'iostream'
class ViewerWidget : public QWidget, public osgViewer::CompositeViewer
{
public:
ViewerWidget(QWidget* parent = 0, Qt::WindowFlags f = 0, osgViewer::ViewerBase::ThreadingModel threadingModel=osgViewer::CompositeViewer::SingleThreaded) : QWidget(parent, f)
{
setThreadingModel(threadingModel);
// disable the default setting of viewer.done() by pressing Escape.
setKeyEventSetsDone(0);
const double r_earth = 6378.137;
const double r_sun = 695990.0;
const double AU = 149697900.0;
// Create the Earth, in blue
osg::ShapeDrawable *earth_sd = new osg::ShapeDrawable;
osg::Sphere* earth_sphere = new osg::Sphere;
earth_sphere-'setName('EarthSphere');
earth_sphere-'setRadius(r_earth);
earth_sd-'setShape(earth_sphere);
earth_sd-'setColor(osg::Vec4(0, 0, 1.0, 1.0));
osg::Geode* earth_geode = new osg::Geode;
earth_geode-'setName('EarthGeode');
earth_geode-'addDrawable(earth_sd);
// Create the Sun, in yellow
osg::ShapeDrawable *sun_sd = new osg::ShapeDrawable;
osg::Sphere* sun_sphere = new osg::Sphere;
sun_sphere-'setName('SunSphere');
sun_sphere-'setRadius(r_sun);
sun_sd-'setShape(sun_sphere);
sun_sd-'setColor(osg::Vec4(1.0, 0.0, 0.0, 1.0));
osg::Geode* sun_geode = new osg::Geode;
sun_geode-'setName('SunGeode');
sun_geode-'addDrawable(sun_sd);
// Move the sun behind the earth
osg::PositionAttitudeTransform *pat = new osg::PositionAttitudeTransform;
pat-'setPosition(osg::Vec3d(0.0, AU, 0.0));
pat-'addChild(sun_geode);
osg::Geometry * unitCircle = new osg::Geometry();
{
osg::Vec4Array * colours = new osg::Vec4Array(1);
(*colours)[0] = osg::Vec4d(1.0,1.0,1.0,1.0);
unitCircle-'setColorArray(colours, osg::Array::BIND_OVERALL);
const unsigned int n_points = 1024;
osg::Vec3Array * coords = new osg::Vec3Array(n_points);
const double dx = 2.0*osg::PI/n_points;
double s,c;
for (unsigned int j=0; j'n_points; ++j) {
s = sin(dx*j);
c = cos(dx*j);
(*coords)[j].set(osg::Vec3d(c,s,0.0));
}
unitCircle-'setVertexArray(coords);
unitCircle-'getOrCreateStateSet()-'setMode(GL_LIGHTING,osg::StateAttribute::OFF);
unitCircle-'addPrimitiveSet(new osg::DrawArrays(osg::PrimitiveSet::LINE_LOOP,0,n_points));
}
osg::Geometry *axes = new osg::Geometry;
{
osg::Vec4Array *colours = new osg::Vec4Array(1);
(*colours)[0] = osg::Vec4d(1.0,0.0,0.0,1.0);
axes-'setColorArray(colours, osg::Array::BIND_OVERALL);
osg::Vec3Array *coords = new osg::Vec3Array(6);
(*coords)[0].set(osg::Vec3d(0.0, 0.0, 0.0));
(*coords)[1].set(osg::Vec3d(0.5, 0.0, 0.0));
(*coords)[2].set(osg::Vec3d(0.0, 0.0, 0.0));
(*coords)[3].set(osg::Vec3d(0.0, 0.5, 0.0));
(*coords)[4].set(osg::Vec3d(0.0, 0.0, 0.0));
(*coords)[5].set(osg::Vec3d(0.0, 0.0, 0.5));
axes-'setVertexArray(coords);
axes-'getOrCreateStateSet()-'setMode(GL_LIGHTING,osg::StateAttribute::OFF);
axes-'addPrimitiveSet(new osg::DrawArrays(osg::PrimitiveSet::LINES,0,6));
}
// Earth orbit
osg::Geode * earthOrbitGeode = new osg::Geode;
earthOrbitGeode-'addDrawable(unitCircle);
earthOrbitGeode-'addDrawable(axes);
earthOrbitGeode-'setName('EarthOrbitGeode');
osg::PositionAttitudeTransform * earthOrbitPAT = new osg::PositionAttitudeTransform;
earthOrbitPAT-'setScale(osg::Vec3d(AU,AU,AU));
earthOrbitPAT-'setPosition(osg::Vec3d(0.0, AU, 0.0));
earthOrbitPAT-'addChild(earthOrbitGeode);
earthOrbitPAT-'setName('EarthOrbitPAT');
osg::Group* scene = new osg::Group;
scene-'setName('SceneGroup');
scene-'addChild(earth_geode);
scene-'addChild(pat);
scene-'addChild(earthOrbitPAT);
QWidget* widget1 = addViewWidget(createGraphicsWindow(0,0,100,100),scene);
QGridLayout* grid = new QGridLayout;
grid-'addWidget( widget1, 0, 0 );
setLayout( grid );
connect( &_timer, SIGNAL(timeout()), this, SLOT(update()) );
_timer.start( 10 );
}
QWidget* addViewWidget( osgQt::GraphicsWindowQt* gw, osg::ref_ptr'osg::Node' scene )
{
osgViewer::View* view = new osgViewer::View;
addView( view );
osg::Camera* camera = view-'getCamera();
camera-'setGraphicsContext( gw );
const osg::GraphicsContext::Traits* traits = gw-'getTraits();
//camera-'setClearColor( osg::Vec4(1.0, 0.0, 0.0, 0.0) );
camera-'setViewport( new osg::Viewport(0, 0, traits-'width, traits-'height) );
camera-'setProjectionMatrixAsPerspective(30.0f, static_cast'double'(traits-'width)/static_cast'double'(traits-'height), 1.0f, 10000.0f );
view-'setSceneData( scene.get() );
view-'addEventHandler( new osgViewer::StatsHandler );
view-'setCameraManipulator( new osgGA::MultiTouchTrackballManipulator );
gw-'setTouchEventsEnabled( true );
return gw-'getGLWidget();
}
osgQt::GraphicsWindowQt* createGraphicsWindow( int x, int y, int w, int h, const std::string& name='Planets', bool windowDecoration=false )
{
osg::DisplaySettings* ds = osg::DisplaySettings::instance().get();
osg::ref_ptr'osg::GraphicsContext::Traits' traits = new osg::GraphicsContext::Traits;
traits-'windowName = name;
traits-'windowDecoration = windowDecoration;
traits-'x = x;
traits-'y = y;
traits-'width = w;
traits-'height = h;
traits-'doubleBuffer = true;
traits-'alpha = ds-'getMinimumNumAlphaBits();
traits-'stencil = ds-'getMinimumNumStencilBits();
traits-'sampleBuffers = ds-'getMultiSamples();
traits-'samples = ds-'getNumMultiSamples();
return new osgQt::GraphicsWindowQt(traits.get());
}
virtual void paintEvent( QPaintEvent* /*event*/ )
{ frame(); }
protected:
QTimer _timer;
};
int main( int argc, char** argv )
{
osg::ArgumentParser arguments(&argc, argv);
#if QT_VERSION '= 0x050000
// Qt5 is currently crashing and reporting 'Cannot make QOpenGLContext current in a different thread' when the viewer is run multi-threaded, this is regression from Qt4
osgViewer::ViewerBase::ThreadingModel threadingModel = osgViewer::ViewerBase::SingleThreaded;
#else
osgViewer::ViewerBase::ThreadingModel threadingModel = osgViewer::ViewerBase::CullDrawThreadPerContext;
#endif
while (arguments.read('--SingleThreaded')) threadingModel = osgViewer::ViewerBase::SingleThreaded;
while (arguments.read('--CullDrawThreadPerContext')) threadingModel = osgViewer::ViewerBase::CullDrawThreadPerContext;
while (arguments.read('--DrawThreadPerContext')) threadingModel = osgViewer::ViewerBase::DrawThreadPerContext;
while (arguments.read('--CullThreadPerCameraDrawThreadPerContext')) threadingModel = osgViewer::ViewerBase::CullThreadPerCameraDrawThreadPerContext;
#if QT_VERSION '= 0x040800
// Required for multithreaded QGLWidget on Linux/X11, see http://blog.qt.io/blog/2011/06/03/threaded-opengl-in-4-8/
if (threadingModel != osgViewer::ViewerBase::SingleThreaded)
QApplication::setAttribute(Qt::AA_X11InitThreads);
#endif
QApplication app(argc, argv);
ViewerWidget* viewWidget = new ViewerWidget(0, Qt::Widget, threadingModel);
viewWidget-'realize();
viewWidget-'setGeometry( 100, 100, 800, 600 );
viewWidget-'show();
return app.exec();
}
[/code]
------------------
Read this topic online here:
http://forum.openscenegraph.org/viewtopic.php?p=70630#70630
_______________________________________________
osg-users mailing list
osg-...@lists.openscenegraph.org
http://lists.openscenegraph.org/listinfo.cgi/osg-users-openscenegraph.org
